DRF Bets Mobile App
This article explores building a mobile betting app backend with Django REST Framework (DRF). We’ll cover setting up the Django project, creating API endpoints for bets, managing user authentication, and deploying the application.
REST API for Mobile Betting Application
A mobile betting app’s functionality hinges on seamless communication with a backend system. This is where a robust REST API built with Django REST Framework (DRF) comes in. DRF, a powerful toolkit for building Web APIs, seamlessly integrates with Django, simplifying the process of creating RESTful APIs. These APIs act as the bridge between your mobile app and the database, handling data requests and responses in a structured and efficient manner.
Here’s a breakdown of how DRF facilitates the creation of your betting app’s API⁚
- Defining API Endpoints⁚ Using DRF, you’ll define clear and concise API endpoints that correspond to various actions users can take within the betting app. For instance, you’d have endpoints for⁚
- Fetching a list of available events to bet on.
- Retrieving detailed information about a specific event.
- Placing a bet with various parameters (e.g., stake, odds).
- Viewing a user’s betting history.
- Data Serialization⁚ DRF’s serializers come into play to translate between the complex Django data models, representing events, bets, and users, and the JSON format that your mobile app can readily understand and work with.
- Handling Authentication and Authorization⁚ Security is paramount in a betting application. DRF provides robust mechanisms for handling user authentication and authorization. This ensures that only authorized users can access specific endpoints and perform actions like placing bets or viewing sensitive information.
By utilizing DRF’s capabilities, you create a well-structured, efficient, and secure REST API that acts as the backbone for your mobile betting application, enabling a smooth and enjoyable user experience.
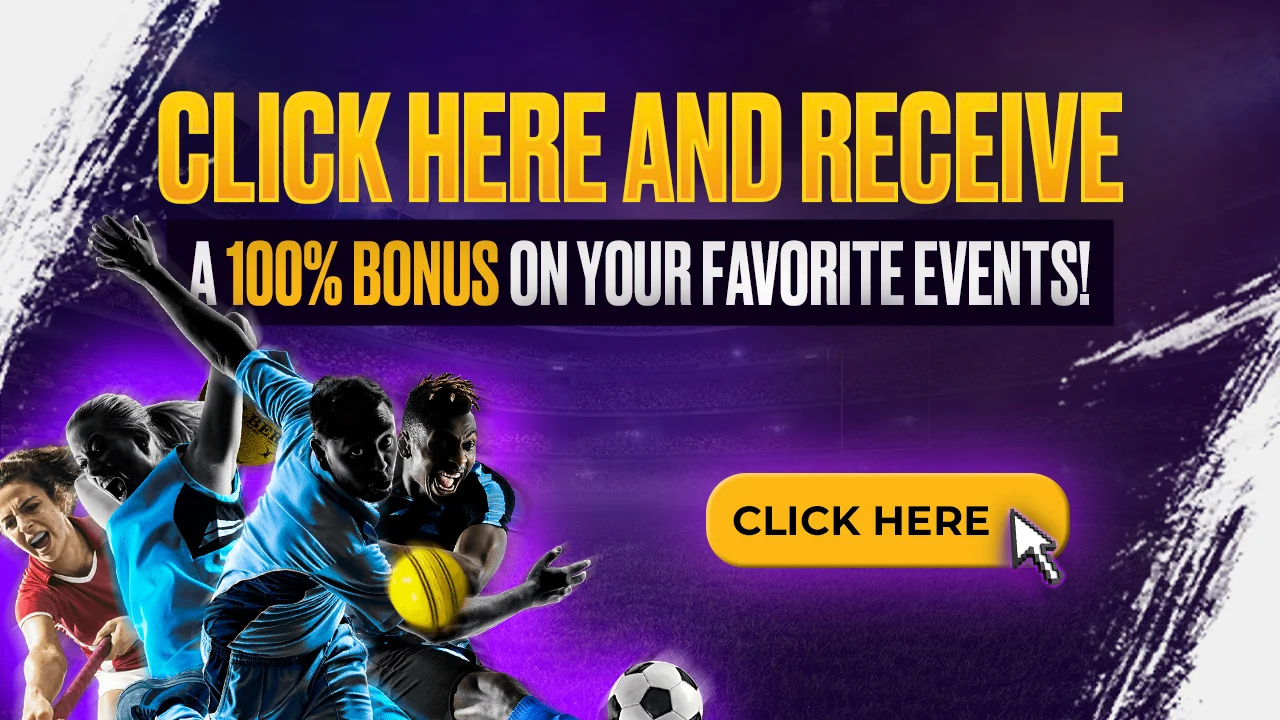
Django Backend for Real-Time Data
In the fast-paced world of mobile betting, real-time data feeds are crucial for providing users with up-to-the-minute odds, event updates, and seamless betting experiences. While Django, at its core, is designed for handling HTTP requests and responses, it can be effectively empowered to manage real-time data flows essential for your mobile app.
Here’s how to equip your Django backend for real-time data handling⁚
- WebSockets with Channels⁚ Django Channels extends Django’s capabilities beyond the standard request/response cycle, enabling persistent connections with clients (your mobile app) using WebSockets. This facilitates pushing real-time updates, such as changing odds or game events, directly to users, enhancing the app’s responsiveness and engagement.
- Asynchronous Task Queues⁚ To prevent blocking real-time updates with long-running tasks like bet processing or external API calls, employ asynchronous task queues like Celery. These queues offload these tasks to background workers, ensuring your Django application remains responsive to WebSocket connections and real-time data handling.
- In-Memory Data Stores⁚ For ultra-fast data retrieval and updates, consider integrating in-memory data stores like Redis alongside your primary database. Redis can cache frequently accessed data, such as live odds or user balances, significantly reducing database load and improving real-time responsiveness.
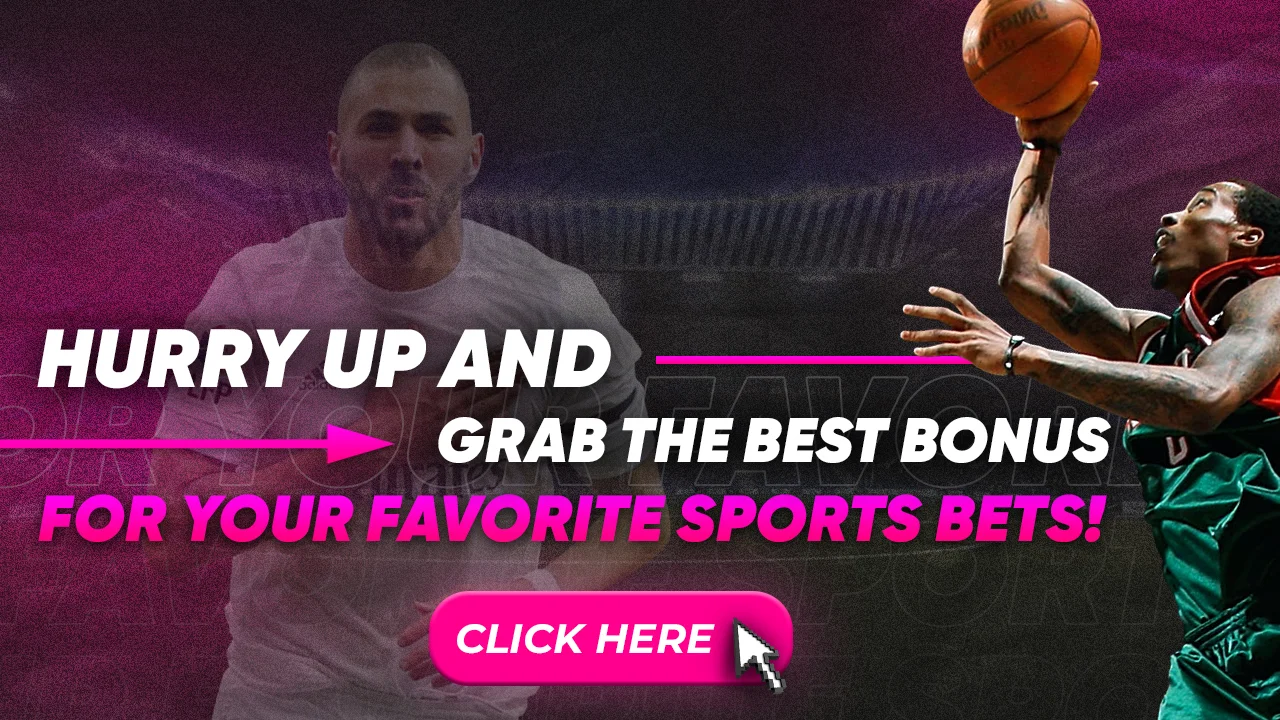
By implementing these strategies, your Django backend transforms into a powerful engine for managing the real-time data demands of your mobile betting app, delivering the dynamic and immediate experiences users expect in the fast-paced world of online betting.
Mobile App Development with React Native and Django
Building a mobile betting app involves creating a seamless and engaging user interface while efficiently communicating with your Django backend. React Native, with its cross-platform capabilities and component-based architecture, emerges as an excellent choice for developing your mobile app front-end.
Here’s how React Native synergizes with your Django backend⁚
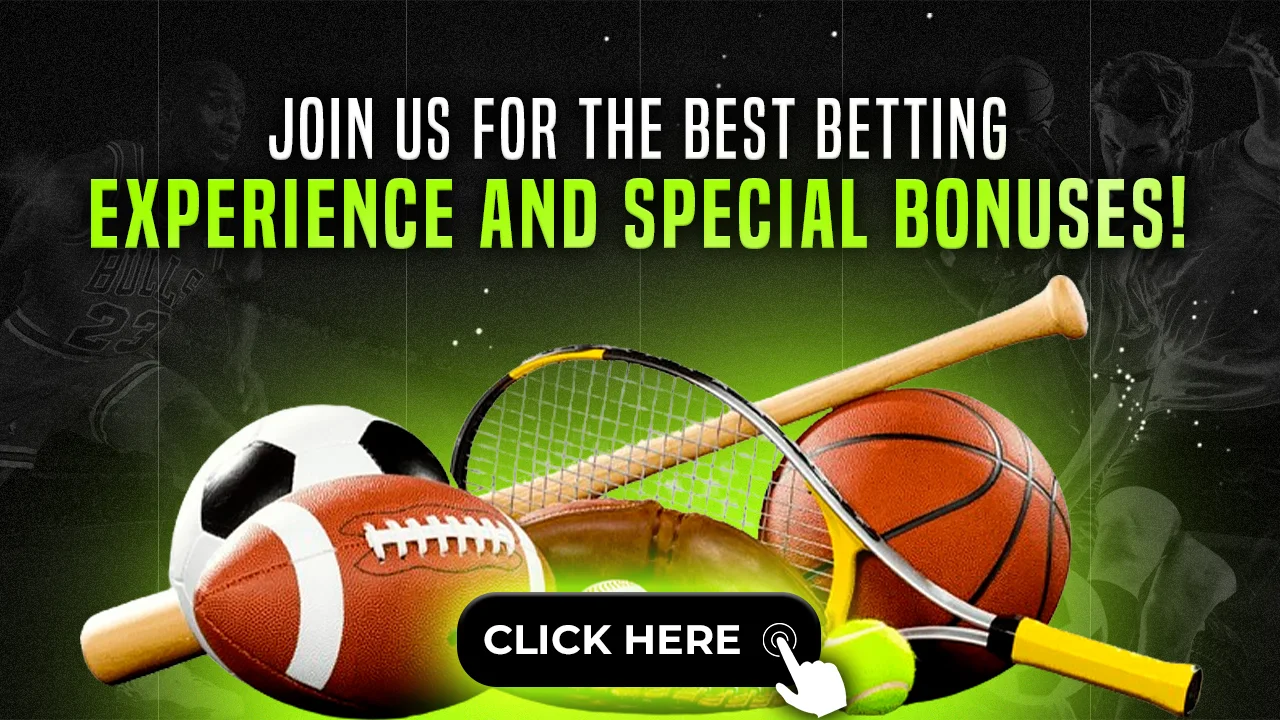
- Communicating with Django REST API⁚ React Native provides libraries like ‘fetch’ or ‘axios’ to easily make API calls to your Django backend. You can retrieve data, such as available bets, odds, or user information, and render it dynamically in your app’s components.
- Real-Time Updates with WebSockets⁚ For real-time features like live odds or bet confirmations, React Native can establish WebSocket connections to your Django backend (powered by Django Channels). This enables pushing instant updates to the app, creating a dynamic and responsive user experience.
- State Management for a Smooth Experience⁚ As users interact with your betting app, managing the application’s state becomes crucial. Libraries like Redux or Context API within React Native help you maintain and update data (e.g., bet selections, user balances) efficiently, ensuring a smooth and consistent UI.
- Cross-Platform Development Efficiency⁚ React Native allows you to build your app for both Android and iOS from a single codebase, significantly reducing development time and effort compared to creating separate native apps.
By choosing React Native for your front-end, you gain access to a powerful and flexible framework that integrates seamlessly with your Django REST API. This combination empowers you to deliver a performant, feature-rich, and engaging mobile betting app to a wider audience.
User Authentication and Security
In a mobile betting application where users handle sensitive financial transactions and personal data, robust authentication and security are paramount. Django REST Framework (DRF) provides a solid foundation for implementing these crucial aspects⁚
- Secure User Registration and Login⁚ DRF offers built-in authentication classes and mixins that simplify the implementation of secure user registration and login workflows. You can leverage these features to handle password hashing, email verification, and secure token-based authentication (e.g., using JWT – JSON Web Tokens).
- Authorization and Permission Control⁚ Distinguish user roles and permissions to control access to specific actions or data. For example, you can restrict bet placement to authenticated users and limit administrative actions to authorized personnel.
- Protecting Sensitive Data During Transmission⁚ Enforce HTTPS for all API communication between your mobile app and the Django backend to encrypt data transmission and prevent eavesdropping. DRF makes it easy to configure your application for HTTPS deployment.
- Data Validation and Sanitization⁚ DRF’s serializers and model validation mechanisms play a vital role in preventing common vulnerabilities like SQL injection or cross-site scripting (XSS). Validate all user input at both the client-side (React Native) and server-side (Django) for an extra layer of protection.
- Rate Limiting and Abuse Prevention⁚ Implement rate limiting on API requests to prevent brute-force attacks or excessive server load. DRF provides throttling classes to easily enforce request limits based on IP addresses or authenticated users.
By meticulously addressing user authentication and security in your DRF Bets mobile app, you foster user trust, safeguard sensitive information, and ensure the integrity of your platform.
Testing and Deployment of Django REST API
Thorough testing and a robust deployment strategy are crucial for delivering a reliable and scalable DRF Bets mobile app. Let’s explore the key aspects⁚
- Comprehensive Testing⁚ Implement a multi-layered testing approach encompassing unit tests, integration tests, and end-to-end tests. Django’s testing framework, along with libraries like pytest, facilitates writing effective tests for your API views, serializers, and business logic. Focus on testing API responses, error handling, and edge cases to ensure correctness.
- Deployment Options⁚ Choose a deployment platform that aligns with your app’s scalability and performance needs. Popular options include cloud providers like AWS, Google Cloud Platform, or Heroku, or self-hosting on virtual private servers (VPS). Consider factors like server location, load balancing, and auto-scaling for optimal user experience.
- Containerization with Docker⁚ Containerize your Django application using Docker to simplify deployment and ensure consistency across different environments. Docker allows you to package your application code, dependencies, and configurations into a portable container, making it easy to deploy and scale.
- Continuous Integration and Continuous Deployment (CI/CD): Implement a CI/CD pipeline to automate the build, testing, and deployment process. Tools like GitLab CI/CD, GitHub Actions, or Jenkins can help you automate these workflows, reducing errors and enabling faster deployments.
- Monitoring and Error Tracking⁚ Once your DRF Bets API is deployed, establish monitoring and error tracking mechanisms. Use tools like Sentry, New Relic, or Datadog to gain insights into API performance, identify bottlenecks, and proactively address errors or exceptions in production.
By prioritizing testing, adopting a suitable deployment strategy, and integrating monitoring, you ensure the stability, reliability, and scalability of your DRF Bets mobile app backend.